Hi Folks,
I have recently written about my Rails Contributions experience
here.
I see that now days contribution is Rails is increased. People love to contribute in Open Source projects. And the way should be easy not painful.
I found that some people are struggling in submitting Pull Requests in Rails on Github. So i thought of writing the same to help them.
Some people are new to git and they don’t know much about git… or sometime patch is very small and they want to use Github’s “fork and edit this file” feature to submit a patch.
And some people who are good in Rails will do it in more easy manner. And easy to open multiple pull request at a time from the same branch.
I am writing some steps to use Github’s fork and edit feature to open pull request.
Open up your project on Github open up file where you want to change
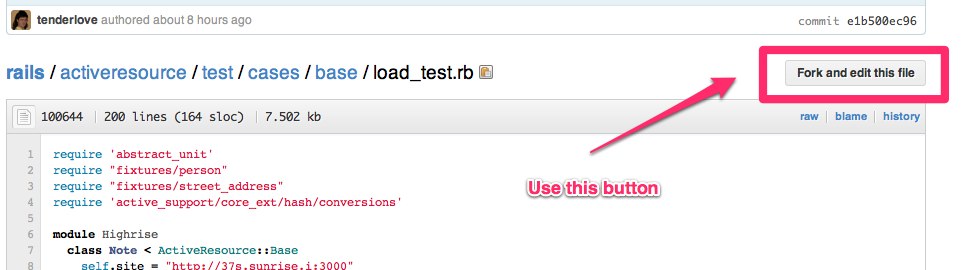
Change your desired things into the file. You can only change one file in one commit
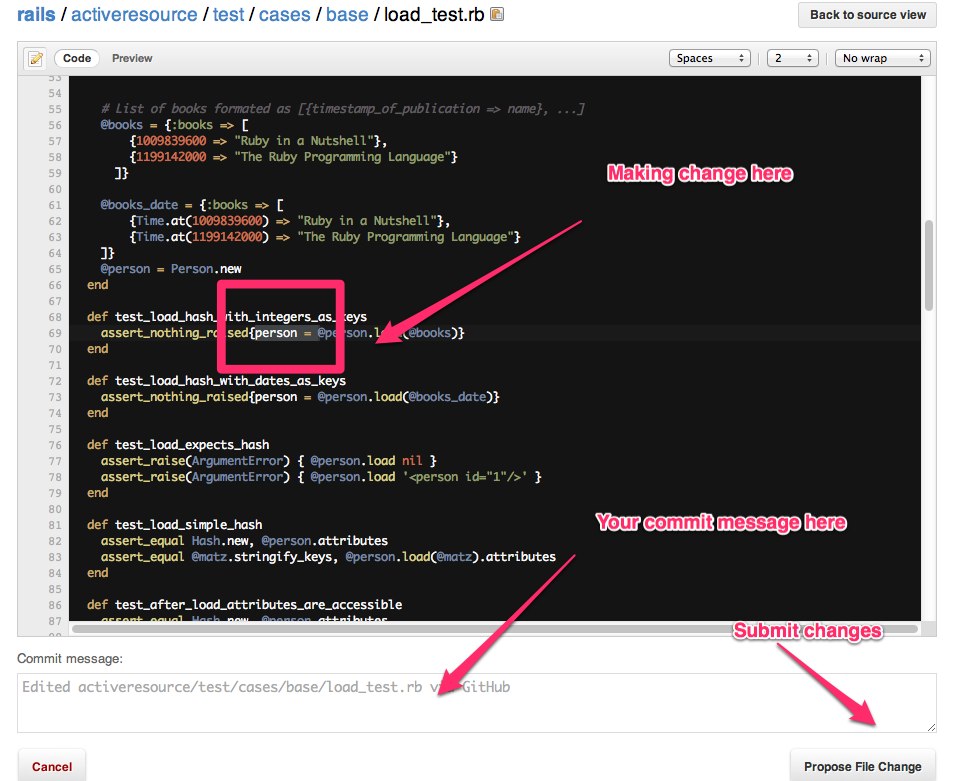
Propese your file changes! Here you can write about your changes. Give some references about issue. Can see the file changed. Can see the commits which you have made.
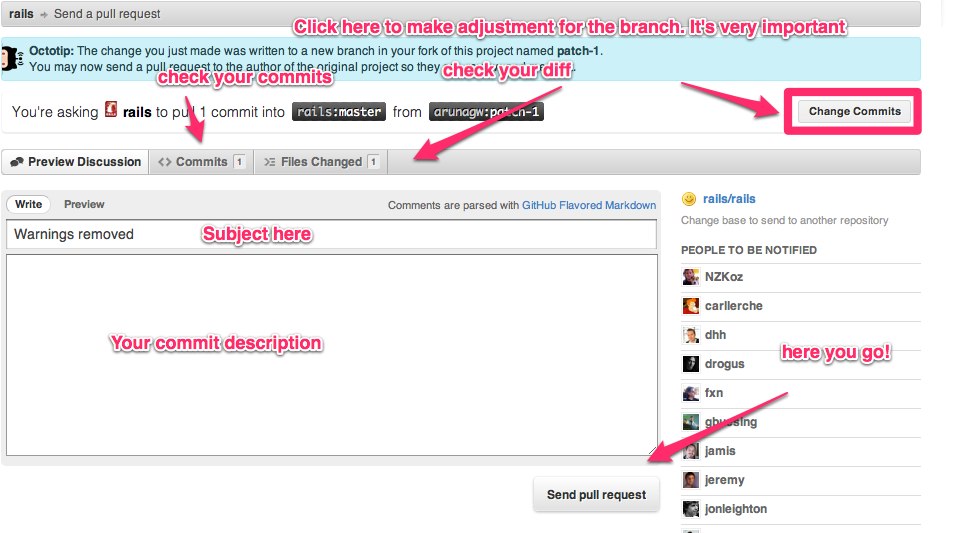
Change commit is the most important part. The reason is when people are doing changes in specific branch. Let’s take a example of Rails. If you are going to submit a patch against Rails 3.0.X version then you must have to choose 3-0-stable branch instead of master in Base branch.
After updating the commit range you can submit the Pull Request and also must see the File Changed.
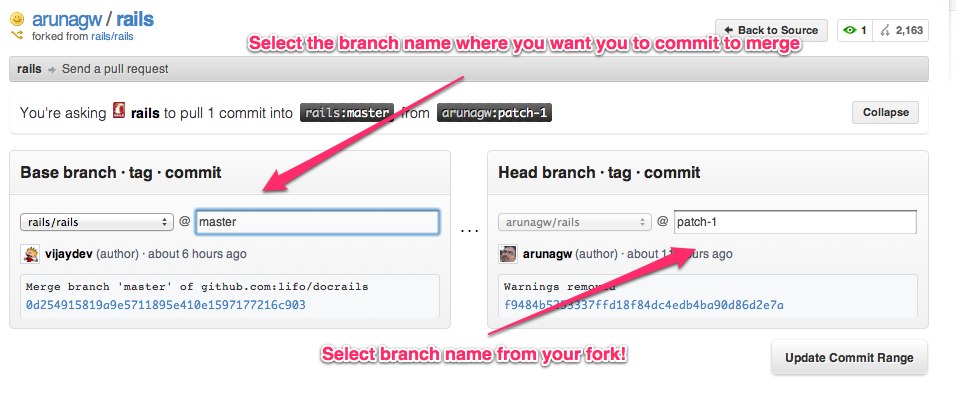
Use this feature when you are fixing any typos, updating any docs. For submitting any code changes you should be running tests. :-)
Feel free to ask me if you face any problem in doing these things. We want more contributions!
You can tweet me @arunagw or catch me on my email any time.
Cheers!